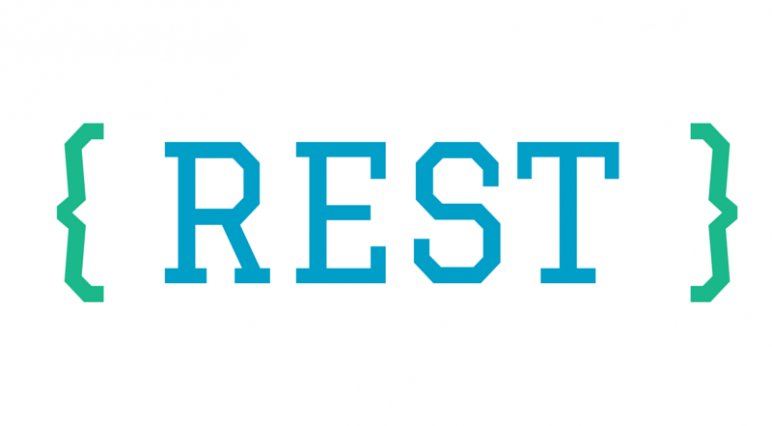
RESTful API endpoints in Drupal can allow you to expose the content of Drupal 7 website to other external resources. These endpoints can be used for fetching data with an AJAX call or simply a web service.
For making complex RESTful web services in Drupal 7, Services module is the best option to go for but if you looking for a simple endpoint in Drupal 7, which outputs JSON response, then going with a custom solution will be the best bet.
Create API endpoint with JSON response in Drupal 7
To create a simple API endpoint in Drupal to serve a JSON put you need to create a custom callback
/**
* Implements hook_menu().
*/
function mymodule_menu() {
$items['api/mymodule'] = array(
'access callback' => true,
'page callback' => 'mymodule_json',
'delivery callback' => 'drupal_json_output',
);
return $items;
}
A noticeable point in this callback is the "delivery callback" argument which runs the output through drupal_json_output function.
/**
* Callback function to return the data.
*/
function mymodule_json() {
$data = ['cat', 'dog', 'mouse', 'elephant'];
return $data;
}
Now if you navigate to the callback url 'api/mymodule' to see the output then you will see the following result
[
"cat",
"dog",
"mouse",
"elephant",
]
Now you can see that this output is exposed as a JSON and can be consumed by other platforms or an AJAX callback to consume data.
The same approach can be used to expose a node or a user as a JSON output.